This is featured post 1 title
Replace these every slider sentences with your featured post descriptions.Go to Blogger edit html and find these sentences.Now replace these with your own descriptions.This theme is Bloggerized by Lasantha - Premiumbloggertemplates.com.
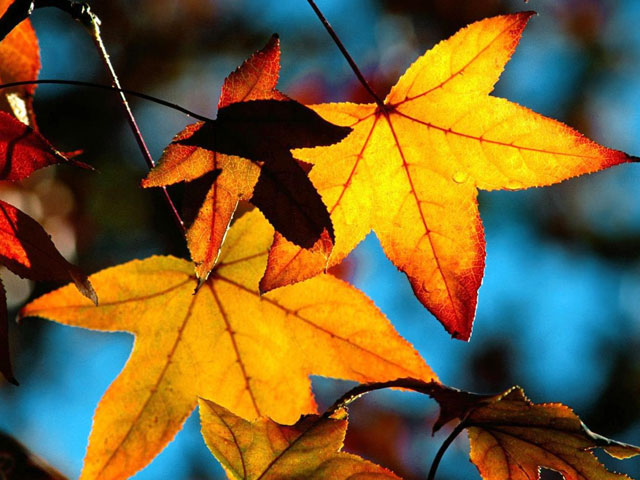
This is featured post 2 title
Replace these every slider sentences with your featured post descriptions.Go to Blogger edit html and find these sentences.Now replace these with your own descriptions.This theme is Bloggerized by Lasantha - Premiumbloggertemplates.com.
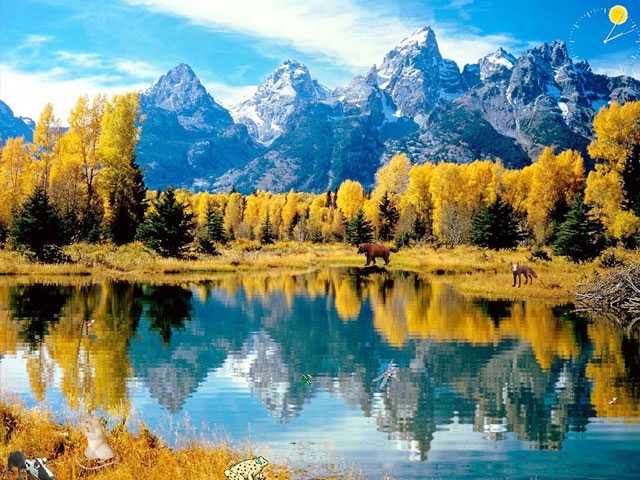
This is featured post 3 title
Replace these every slider sentences with your featured post descriptions.Go to Blogger edit html and find these sentences.Now replace these with your own descriptions.This theme is Bloggerized by Lasantha - Premiumbloggertemplates.com.
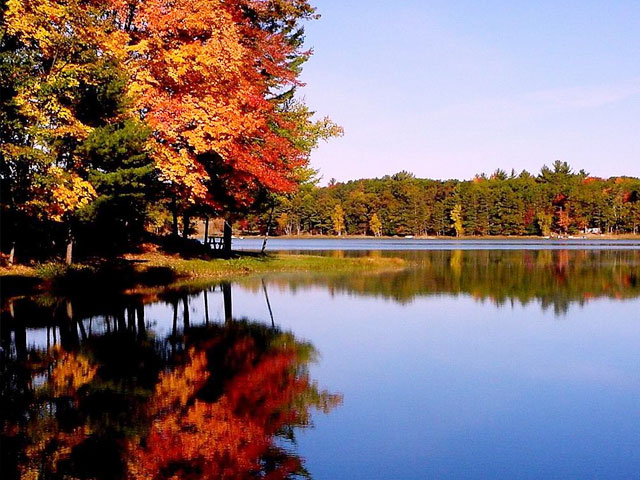
Monday, December 20, 2010
Saturday, December 18, 2010
User-defined Functions:gcufbioinfo


reverse
? >>> def reverse(s):
... """Return the sequence string in reverse order."""
... letters = list(s)
... letters.reverse()
... return ''.join(letters)
...
>>> reverse('CCGGAAGAGCTTACTTAG')
'GATTCATTCGAGAAGGCC'
There are a few new things in this function that need explanation. First, we've used an argument name of "s
" instead of "dna
". You can name your arguments whatever you like in Python. It is something of a convention to use short names based on their expected value or meaning. So "s
" for string is fairly common in Python code. The other reason to use "s
" instead of "dna
" in this example is that this function works correctly on any string, not just strings representing dna sequences. So "s
" is a better reflection of the generic utility of this function than "dna
". You can see that the
reverse
function takes in a string, creates a list based on the string, and reverses the order of the list. Now we need to put the list back together as a string so we can return a string. Python string objects have a join()
method that joins together a list into a string, separating each list element by a string value. Since we do not want any character as a separator, we use the join()
method on an empty string, represented by two quotes (''
or ""
).User-defined Functions:gcufbioinfo


def
, is followed by the name of the function and any arguments (expected input values) surrounded by parentheses, and ends with a colon. Subsequent lines make up the body of the function and must be indented. If a string comment appears in the first line of the body, it becomes part of the documentation for the function. The last line of a function returns a result.Let's define some functions in the PyCrust shell. Then we can try each function with some sample data and see the result returned by the function.
>>> def transcribe(dna):
... """Return dna string as rna string."""
... return dna.replace('T', 'U')
...
>>> transcribe('CCGGAAGAGCTTACTTAG')
'CCGGAAGAGCUUACUUAG'
Python Functions:gcufbioinfo


len()
returns the number of items in a sequence; dir()
returns a list of strings representing the attributes of an object; list()
returns a new list initialized from some other sequence. >>> dna = 'CTGACCACTTTACGAGGTTAGC'
>>> bases = ['A', 'C', 'G', 'T']
>>> len(dna)
22
>>> len(bases)
4
>>> dir(dna)
['__add__', '__class__', '__contains__', '__delattr__',
'__doc__', '__eq__', '__ge__', '__getattribute__', '__getitem__',
'__getslice__', '__gt__', '__hash__', '__init__', '__le__',
'__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__',
'__repr__', '__rmul__', '__setattr__', '__str__', 'capitalize',
'center', 'count', 'decode', 'encode', 'endswith', 'expandtabs',
'find', 'index', 'isalnum', 'isalpha', 'isdigit', 'islower',
'isspace', 'istitle', 'isupper', 'join', 'ljust', 'lower',
'lstrip', 'replace', 'rfind', 'rindex', 'rjust', 'rstrip', 'split',
'splitlines', 'startswith', 'strip', 'swapcase', 'title',
'translate', 'upper']
>>> dir(bases)
['__add__', '__class__', '__contains__', '__delattr__',
'__delitem__', '__delslice__', '__doc__', '__eq__', '__ge__',
'__getattribute__', '__getitem__', '__getslice__', '__gt__',
'__hash__', '__iadd__', '__imul__', '__init__', '__le__', '__len__',
'__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__repr__',
'__rmul__', '__setattr__', '__setitem__', '__setslice__', '__str__',
'append', 'count', 'extend', 'index', 'insert', 'pop', 'remove',
'reverse', 'sort']
>>> list(dna)
['C', 'T', 'G', 'A', 'C', 'C', 'A', 'C', 'T', 'T', 'T',
'A', 'C', 'G', 'A', 'G', 'G', 'T', 'T', 'A', 'G', 'C']
Python Lists:gcufbioinfo


>>> bases = ['A', 'C', 'G', 'T']
>>> bases
['A', 'C', 'G', 'T']
>>> bases.append('U')
>>> bases
['A', 'C', 'G', 'T', 'U']
>>> bases.reverse()
>>> bases
['U', 'T', 'G', 'C', 'A']
>>> bases[0]
'U'
>>> bases[1]
'T'
>>> bases.remove('U')
>>> bases
['T', 'G', 'C', 'A']
>>> bases.sort()
>>> bases
['A', 'C', 'G', 'T']
In this example we created a list of single characters that we called bases. Then we added an element to the end, reversed the order of all the elements, retrieved elements by their index position, removed an element with the value'U'
, and sorted the elements. Removing an element from a list illustrates a situation where we need to supply theremove()
method with an additional piece of information, namely the value that we want to remove from the list. As you can see in the picture below, PyCrust takes advantage of Python's ability to let us know what is required for most
operations by displaying that information in a call tip pop-up window.
Python Strings:gcufbioinfo


'
), double ("
) or triple ('''
or """
) quotes. In the example we assigned the string literal CTGACCACTTTACGAGGTTAGC
to the variable named dna
. >>> dna = 'CTGACCACTTTACGAGGTTAGC'
Then we simply typed the name of the variable, and Python responded by displaying the value of that variable, surrounding the value with quotes to remind us that the value is a string. >>> dna
'CTGACCACTTTACGAGGTTAGC'
A Python string has several built-in capabilities. One of them is the ability to return a copy of itself with all lowercase letters. These capabilities are known as methods. To invoke a method of an object, use the dot syntax. That is, you type the name of the variable (which in this case is a reference to a string object) followed by the dot (.
) operator, then the name of the method followed by opening and closing parentheses.>>> dna.lower()
'ctgaccactttacgaggttagc'
You can access part of a string using the indexing operator s[i]
. Indexing begins at zero, so s[0]
returns the first character in the string, s[1]
returns the second, and so on.>>> dna[0]
'C'
>>> dna[1]
'T'
>>> dna[2]
'G'
>>> dna[3]
'A'
The final line in our screen shot shows PyCrust's autocompletion feature, whereby a list of valid methods (and properties) of an object are displayed when a dot is typed following an object variable. As you can see, Python lists have many built-in capabilities that you can experiment with in the Python shell. Now let's look at one of the other Python sequence types, the list.Beginning Python for Bioinformatics:gcufbioinfo


What I concluded:


Predicting Breast Cancer Survivability Using Data Mining Techniques


Combined Supervised and Unsupervised Learning in Genomic Data Mining


Acute Coronary Syndrome Prediction Using Data Mining Techniques- An Application


Biological Data Mining for Genomic Clustering Using Unsupervised Neural Learning:


Quratt ul ain Siddique
Data Mining and Visualization of Mouse Genome Data:


Quratt ul ain Siddique
Saturday, November 27, 2010
Rasmol:gcufbioinfo


Rasmol:gcufbioinfo


click Display>and select different options to view your molecule.
Rasmol:gcufbioinfo


And save this file to any directory with file format ".pdb" so now you can visualize already saved molecule.
Rasmol:gcufbioinfo


enter protein name for which you want to view the structure. As I entered DNA Polymerase so the result is http://www.pdb.org/pdb/explore/explore.do?structureId=3OGU
the out put is as:
At the right of this page you can there is the option of Download files so click this. After clicking this select "PDB files(gz)".then download and install winzip to your system and download this file automatically to raswin and view the structure in rasmol.
What kinds of molecules can RasMol display?:Gcufbioinfo


In order to display a molecule, RasMol needs a data file called an atomic coordinate file. This data file specifies the position of every atom in the molecule, as cartesian coordinates X, Y, and Z.
Three-dimensional structures can be predicted for many small molecules, but must be determined empirically for macromolecules. The most common method for determining structure is X-ray diffraction analysis of a crystal. Nuclear magnetic resonance (NMR) can also be used. Some structures are available only as theoretical models, often based on related molecules for which empirical structures have been determined.
Rasmol Tool:


here. Install Rasmol to your system and get started.
Thursday, November 25, 2010
HTML Calculator:gcufbioinfo


function sqrt(form)
{
form.displayvalue.value=Math.sqrt(form.displayvalue.value);
}
function sq(form)
{
form.displayvalue.value=eval(form.displayvalue.value)*eval(form.displayvalue.value);
}
function ln(form)
{
form.displayvalue.value=Math.exp(form.displayvalue.value);
}
function equal(form)
{
form.displayvalue.value=eval(form.displayvalue.value);
}
function delChar(input)
{
input.value=input.value.substring(0,input.value-length-1);
}
function addChar(input,character)
{
if(input.value==null||input.value=="0")
input.value=character
else
input.value+=character
}
Insha Allah you will see your functioning very soon regards Admin Quratt ul ain Siddique.
Monday, November 22, 2010
HTML Calculator:gcufbioinfo


<head>
<script type="text/javascript">
function cos(form)
{
form.displayvalue.value=Math.cos(form.displayvalue.value);
}
function sin(form)
{
form.displayvalue.value=Math.sin(form.displayvalue.value);
}
function tan(form)
{
form.displayvalue.value=Math.tan(form.displayvalue.value);
}
</script>
</head>
I will tell you other codes as soon as possible and you will see Insha Allah your calculator functional
HTML Calculator:gcufbioinfo


<html>
<title>Calculator</title>
<head></head>
<body>
<table border="2">
<tr>
<td><input name="displayvalue" size="39" value="0"></td>
<tr>
<td>
<table border="2">
<tr>
<td>
<input type="button" value=" char ">
</td>
<td>
<input type="button" value=" delete ">
</td>
<td>
<input type="button" value=" = ">
</td>
</tr>
</table>
</td>
<tr>
<td>
<table border="2">
<tr>
<td>
<input type="button" value=" exp ">
</td>
<td>
<input type="button" value=" 7 ">
</td>
<td>
<input type="button" value=" 8 ">
</td>
<td>
<input type="button" value=" 9 ">
</td>
<td>
<input type="button" value=" / ">
</td>
</tr>
<tr>
<td>
<input type="button" value=" ln ">
</td>
<td>
<input type="button" value=" 4 ">
</td>
<td>
<input type="button" value=" 5 ">
</td>
<td>
<input type="button" value=" 6 ">
</td>
<td>
<input type="button" value=" * ">
</td>
</tr>
<tr>
<td>
<input type="button" value=" sqrt ">
</td>
<td>
<input type="button" value=" 1 ">
</td>
<td>
<input type="button" value=" 2 ">
</td>
<td>
<input type="button" value=" 3 ">
</td>
<td>
<input type="button" value=" - ">
</td>
</tr>
<tr>
<td>
<input type="button" value=" sq ">
</td>
<td>
<input type="button" value=" 0 ">
</td>
<td>
<input type="button" value=" . ">
</td>
<td>
<input type="button" value=" % ">
</td>
<td>
<input type="button" value=" + ">
</td>
</tr>
<tr>
<td>
<input type="button" value=" ( ">
</td>
<td>
<input type="button" value=" cos ">
</td>
<td>
<input type="button" value=" sin ">
</td>
<td>
<input type="button" value=" tan ">
</td>
<td>
<input type="button" value=" ) ">
</td>
</tr>
</table>
</td>
</tr>
</tr>
</tr>
</table>
</body>
</html>
out put is as:
Now I tell you how to make it functional
Quratt ul ain Siddique
Sunday, November 21, 2010
imageJ:gcufbioinfo


Medical Image Processing tool:


First save an image especially a medical image to your system and copy this image and go to C derive then program files and then imagej folder and paste this image into it. then open imageJ from start menu and click file >open as shown in gcufbioinfo and select the picture to view.
you can view your image using this tool .
imageJ:gcufbioinfo


Download ImageJ:


the page will open
click download
http://rsbweb.nih.gov/ij/download.html
then get latest version of imageJ for your OS . If you are new to use ImageJ then Download ImageJ 1.43 bundled with 32-bit Java 1.6.0_10 (25MB) other wise download without java for windows OS.
Medical Image Processing tool:


ImageJ is a public domain, Java-based image processing program developed at the National Institutes of Health. ImageJ was designed with an open architecture that provides extensibility via Java plugins and recordable macros. Custom acquisition, analysis and processing plugins can be developed using ImageJ's built-in editor and a Java compiler. User-written plugins make it possible to solve many image processing and analysis problems, from three-dimensional live-cell imaging, to radiological image processing,multiple imaging system data comparisonsto automated hematology systems.ImageJ's plugin architecture and built in development environment has made it a popular platform for teaching image processing.
Tuesday, November 2, 2010
primary structure analysis:


Radar:De novo repeat detection in protein sequences
I entered the protein albumin in uniprot and get the following result It gave the number of repeats present in the sequence.
http://www.ebi.ac.uk/Tools/es/cgi-bin/jobresults.cgi/radar/radar-20101102-1505421645.html
Monday, November 1, 2010
primary structure analysis


Radar :De novo repeat detection in protein sequences go to http://www.ebi.ac.uk/Tools/Radar/index.html
primary structure analysis:


HeliQuest A web server to screen sequences with specific alpha-helical properties HeliQuest calculates from an α-helix sequence its physicochemical properties and amino acid composition and uses the results to screen any databank in order to identify protein segments possessing similar features.
The server is divided into 2 interconnected modules: the sequence analysis module and the screening module.
In addition, the mutation module, (available from the sequence analysis module), allows user to mutate helices manually or automatically by genetic algorithm to create analogues with specific properties.-http://heliquest.ipmc.cnrs.fr/
After entering the helix sequence of the protein this protein sequence is get by entering the name of the protein in uniprot then paste it by clicking the analysis button and get the following
http://heliquest.ipmc.cnrs.fr/cgi-bin/ComputParams.py


ScanSite pI/Mw:Compute the theoretical pI and Mw, and multiple phosphorylation states. Enter a protein name and sequence below. The molecular weight and isoelectric point of this sequence and multiple phosphorylation states will be displayed. http://scansite.mit.edu/calc_mw_pi.html
out put will be
primary structure analysis 3:


Compute pI/Mw: Compute the theoretical isoelectric point (pI) and molecular weight (Mw) from a UniProt Knowledgebase entry or for a user sequence
now go to this tool and get the required result Please enter one or more UniProtKB/Swiss-Prot protein identifiers (ID) (e.g. ALBU_HUMAN) or UniProt Knowledgebase accession numbers (AC) (e.g. P04406), separated by spaces, tabs or newlines. Alternatively, enter a protein sequence in single letter code. The theoretical pI and Mw (molecular weight) will then be computed. previosly I entered the amino acid sequence and now I am going to enter the uniprot AC#.http://www.expasy.org/cgi-bin/pi_tool
it will give you this format of result so click on the following things according to the instructions mentioned Please select one of the following features by clicking on a pair of endpoints, and the computation will be carried out for the corresponding sequence fragment.
Primary structure analysis 2:


ProtParam :Physico-chemical parameters of a protein sequence (amino-acid and atomic compositions, isoelectric point, extinction coefficient, etc.)
First go to this page http://www.expasy.org/tools/ select Primary structure analysis and select the first tool as I mentioned. click the tool and I entered the amino acid sequence of protein transgelin-2 in http://www.expasy.org/tools/protparam.html then get the out put page
http://www.expasy.org/cgi-bin/protparam
the out put is
Primary structure analysis:


I will breifly tell you how to use these tools.
HTML Calculator:


<input type="button" value="submit">
<html>
<body>
<input type="button" value="submit">
<br><br>
<table border="2">
<tr><td><input type="text"></td></tr>
<tr><table border="2"><tr><td><input type="button" value="1"></td><td><input type="button" value="2"></td><td><input type="button" value="3"></td><td><input type="button" value="c"></td></tr><tr><td><input type="button" value="4"></td><td><input type="button" value="5"></td><td><input type="button" value="6"></td><td><input type="button" value="/"></td></tr><tr><td><input type="button" value="7"></td><td><input type="button" value="8"></td><td><input type="button" value="9"></td><td><input type="button" value="*"></td></tr><tr><td><input type="button" value="0"></td><td><input type="button" value="."></td><td><input type="button" value="="></td><td><input type="button" value="-"></td></tr><tr><td><input type="button" value="+"></td><td><input type="button" value="%"></td><td><input type="button" value="d"></td><td><input type="button" value="f"></td></tr></table></tr>
</table>
</body>
</html>
Saturday, October 30, 2010
Muliple Sequence Alignment


http://www.ebi.ac.uk/Tools/es/cgi-bin/clustalw2/result.cgi?tool=clustalw2&jobid=clustalw2-20101030-1758594671&poll=yes
This is the multiple sequence alignment of the selected sequences
Muliple Sequence Alignment


enter your query for which you want to retrieve the sequences
or you can also go to uniprot which in my opinion is easy to use and get fasta sequence so go to uniprot and enter protein hemoglobin and get different entries of this protein click them and get fasta sequence like this
and select many proteins and get their fasta formats like this
click on fasta and http://www.uniprot.org/uniprot/P68871.fasta
copy and paste these fasta sequences of many proteins in a text file and collect all these sequences in a text document.
In this format I pasted my all sequences in my notepad
ClustalW:


PDB structures part 3


http://www.ncbi.nlm.nih.gov/Structure/mmdb/mmdbsrv.cgi?uid=34605
get this PDB ID and entered into PDB and get the same 3D structure of the protein
http://www.rcsb.org/pdb/explore/explore.do?structureId=1WYM
PDB structures part 2


the protein entered is Transgelin-2
http://www.ncbi.nlm.nih.gov/Structure/mmdb/mmdbsrv.cgi?uid=34605
view 3D structure using cn3d which is a structure viewer I mentioned in my very previous tutorial how to use and download cn3d 4.0 to your systems
PDB Structures


I select three Tumor Supressor Genes
1.
p16INK4a
2.
p53
3.
RB - the retinoblastoma gene
entered these genes in ncbi http://www.ncbi.nlm.nih.gov/
select nucleotide in search and enter the gene name
p16INK4a get this page http://www.ncbi.nlm.nih.gov/nuccore/NM_001001522.1
3.PDB:


How to Search PDB:
go to http://www.rcsb.org/pdb/explore/motm.do
enter PDB ID if you don't know the ID then enter the text methylenetetrahydrofolate reductase to get the structural information of that protein
I entered this text then get this result go to this page http://www.rcsb.org/pdb/results/results.do?outformat=&qrid=FA02BFE5&tabtoshow=Current
Friday, October 29, 2010
2. PUBCHEM:


if you enter chlorpropamide which is the molecule use for the treatment of Type II diebetes it will give complete information about the structure and function of the molecule.
PubChem provides information on the biological activities of small molecules. It is a component of NIH's Molecular Libraries Roadmap Initiative.
visit this page I have entered the molecule name
http://pubchem.ncbi.nlm.nih.gov/summary/summary.cgi?cid=2727&loc=ec_rcs
Out put:


and it gives the results that you get by accessing this page
http://www.drugbank.ca/drugs/DB00672
1. LIGAND PREPARATION:


a. Ligands Collection:
1. DRUGBANK :http://www.drugbank.ca/
first go to this link enter your protein or condition like any disease and disorder it will give you complete information about the drug which is designed for the particular purpose eg enter anxiety disorder in drugbank it will give you different ligands of that particular disorder and protein and also gives complete information about the target protein.
The DrugBank database is a unique bioinformatics and cheminformatics resource that combines detailed drug (i.e. chemical, pharmacological and pharmaceutical) data with comprehensive drug target (i.e. sequence, structure, and pathway) information. The database contains nearly 4800 drug entries including >1,480 FDA-approved small molecule drugs, 128 FDA-approved biotech (protein/peptide) drugs, 71 nutraceuticals and >3,200 experimental drugs. Additionally, more than 2,500 non-redundant protein (i.e. drug target) sequences are linked to these FDA approved drug entries. Each DrugCard entry contains more than 100 data fields with half of the information being devoted to drug/chemical data and the other half devoted to drug target or protein data.
Steps Involved in Drug Designing


I. LIGAND PREPARATION
II. RECEPTOR PREPARATION
III. DOCKING
IV. BINDING AFFINITY STUDIES
DRUG DESIGNING


he shortcoming of traditional drug discovery; as well as the allure of a more deterministic approach to combating disease has led to the concept of "Rational drug design" (Kuntz 1992). Nobody could design a drug before knowing more about the disease or infectious process than past. For "rational" design, the first necessary step is the identification of a molecular target critical to a disease process or an infectious pathogen. Then the important prerequisite of "drug design" is the determination of the molecular structure of target, which makes sense of the word "rational".
In fact, the validity of "rational" or "structure-based"drug discovery rests largely on a high-resolution target structure of sufficient molecule detail to allow selectivity in the screening of compounds. Simple flowchart for drug designing shown in the figure:
Monday, October 11, 2010
HTML Frames:


<html>
<frameset rows="20%,80%">
<frame src="file:///E:/anny.html" name="frame1" noresize="noresize">
<frame src="http://www.islamickorner.com">
</frameset>
</html>
one new thing is noresize attribute which means you can not resize your page as previously by dragging its size is fixed you can check it by dragging your page it can't be happened.
the out put is as:
HTML Images2:


out put is as:
click the second image to get the next page

<a href="http://www.bermuda-online.org/roses2.gif"><img src="file:///C:/Documents%20and%20Settings/Administrator/My%20Documents/My%20Pictures/roses2.gif" height="200" width="200" border="10"></img></a>